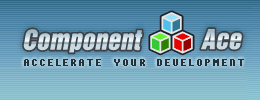 |
|
|
 |
Here is the list of error codes which could be useful to handle errors and to provide user with the proper error message.
You can see a practical example of errors handling in the MultiUser demo supplied with the Absolute DB.
// error codes
const ABS_ERR_OK = 0;
const ABS_ERR_INSERT_RECORD = -1;
const ABS_ERR_UPDATE_RECORD = -2;
const ABS_ERR_DELETE_RECORD = -3;
const ABS_ERR_UPDATE_RECORD_MODIFIED = -4;
const ABS_ERR_DELETE_RECORD_MODIFIED = -5;
const ABS_ERR_UPDATE_RECORD_DELETED = -6;
const ABS_ERR_DELETE_RECORD_DELETED = -7;
const ABS_ERR_CONSTRAINT_VIOLATED = -8;
const ABS_ERR_UPDATE_RECORD_PROHIBITED = -9;
const ABS_ERR_DELETE_RECORD_PROHIBITED = -10;
const ABS_ERR_CANCEL_PROHIBITED = -11;
const ABS_ERR_RECORD_LOCKED = -12;
const ABS_ERR_TABLE_LOCKED = -13;
Example:
procedure TMainForm.ABSTable1DeleteError(DataSet: TDataSet;
E: EDatabaseError; var Action: TDataAction);
begin
Action:=daAbort;
if (E is EABSEngineError) then
case (EABSEngineError(E).ErrorCode) of
ABS_ERR_RECORD_LOCKED:
begin
if MessageDlg('The record is locked. '+
'Do you want to try to delete this record again?',
mtWarning,[mbYes,mbNo],0)=mrYes then
Action:=daRetry;
end;
ABS_ERR_TABLE_LOCKED:
begin
if MessageDlg('The table is locked. '+
'Do you want to try to delete this record again?',
mtWarning,[mbYes,mbNo],0)=mrYes then
Action:=daRetry;
end;
ABS_ERR_DELETE_RECORD_MODIFIED:
begin
MessageDlg('The record you are trying to delete has been modified by another user. '+
'The table will now be refreshed. If you want to delete this record, try again.',
mtWarning,[mbOk],0);
DataSet.Refresh;
end;
ABS_ERR_DELETE_RECORD_DELETED:
begin
MessageDlg('The record you are trying to delete has been deleted by another user '+
'The table will now be refreshed',
mtWarning,[mbOk],0);
DataSet.Refresh;
end
else
MessageDlg(E.Message,mtError,[mbOK],0);
end
else
MessageDlg(E.Message,mtError,[mbOK],0);
end;
|
|